Knowing multiple ways on how to manipulate arrays in Python is an important step towards efficiently writing code. Here are some common ways to manipulate arrays in Python:
Indexing: Access individual elements of the array by their index, starting from 0. Example:
arr = [1, 2, 3]
print(arr[1])
# outputs 2
PythonSlicing: Access a sub-section of the array by specifying start and end indices. Example:
arr = [1, 2, 3, 4, 5]
print(arr[1:3])
# outputs [2, 3]
Python
Modifying values: Change individual elements of the array by assigning new values to them using their indices. Example:
arr = [1, 2, 3]
arr[1] = 4
# print(arr) outputs [1, 4, 3]
PythonAppending: Add elements to the end of the array using the append() method. Example:
arr = [1, 2, 3]
arr.append(4)
print(arr)
# outputs [1, 2, 3, 4]
PythonInserting: Add elements at specific indices using the insert() method. Example:
arr = [1, 2, 3]
arr.insert(1, 4)
print(arr)
# outputs [1, 4, 2, 3]
PythonDeleting: Remove elements from the array using the remove() method or del keyword. Example:
arr = [1, 2, 3]
arr.remove(2)
print(arr)
# outputs [1, 3]
PythonConcatenating: Combine two arrays into a single array using the + operator. Example:
arr1 = [1, 2]
arr2 = [3, 4]
arr3 = arr1 + arr2
print(arr3)
# outputs [1, 2, 3, 4]
PythonNote that Python has a built-in list data structure which is dynamic array and can be used for these operations.
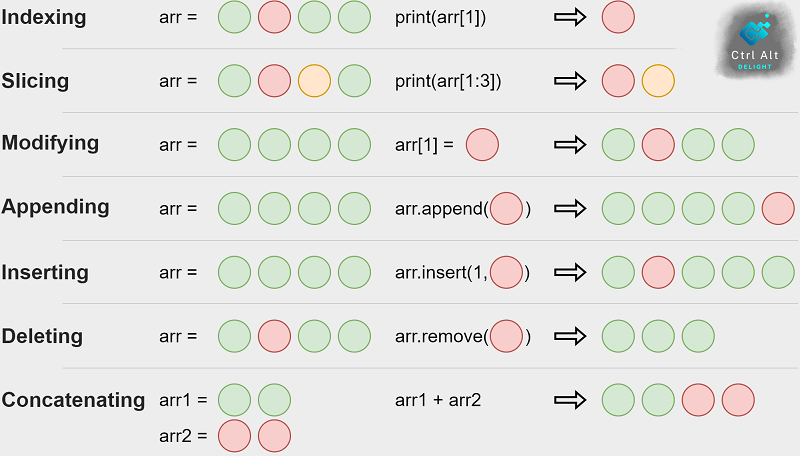