Understanding collider events is crucial for creating interactive and dynamic games. In Unity, a collider is a component that can be attached to a game object to detect collisions with other game objects. Collider events are events that are triggered when a collider intersects or collides with another collider.
Types of Collider Events
Here is a table of all the different collider events in Unity:
Event | Description |
---|---|
OnCollisionEnter | Triggered when a collision first occurs |
OnCollisionStay | Triggered while a collision is ongoing |
OnCollisionExit | Triggered when a collision ends |
OnTriggerEnter | Triggered when a collider enters a trigger collider |
OnTriggerStay | Triggered while a collider is within a trigger collider |
OnTriggerExit | Triggered when a collider exits a trigger collider |
Add a Collider
To add a collider component to a game object in Unity, you can follow either of the two methods:
- Click on the game object in the scene view, then in the inspector window, click “Add Component” and select “Collider” from the menu.
- Click on the game object in the hierarchy view, then in the inspector window, click “Add Component” and select “Collider” from the menu.
Here is an example of adding a collider component to a game object:
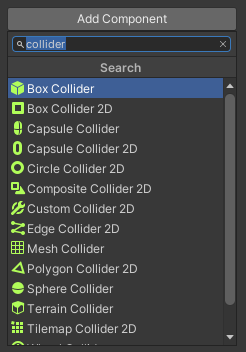
The “is Trigger” option
When both objects have colliders, collider events are called. The “is trigger” option allows you to trigger collision events without doing physical collisions, which is useful for checking whether two objects’ colliders overlap.
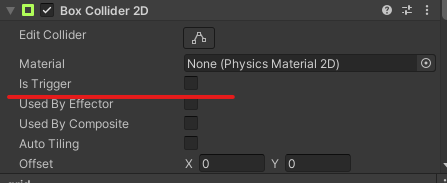
Defining the Event in C#
Let’s take a look at an example of how to use collider events in Unity. Suppose we have a game object with a collider component attached to it, and we want to destroy it when it collides with another game object with a tag of “Obstacle”. We can use the OnCollisionEnter event to detect the collision and then check the other collider’s tag to see if it’s an obstacle. Here’s what the code would look like:
private void OnCollisionEnter(Collision collision)
{
if(collision.gameObject.CompareTag("Obstacle"))
{
Destroy(gameObject);
}
}
C#Defining which objects collide with each other
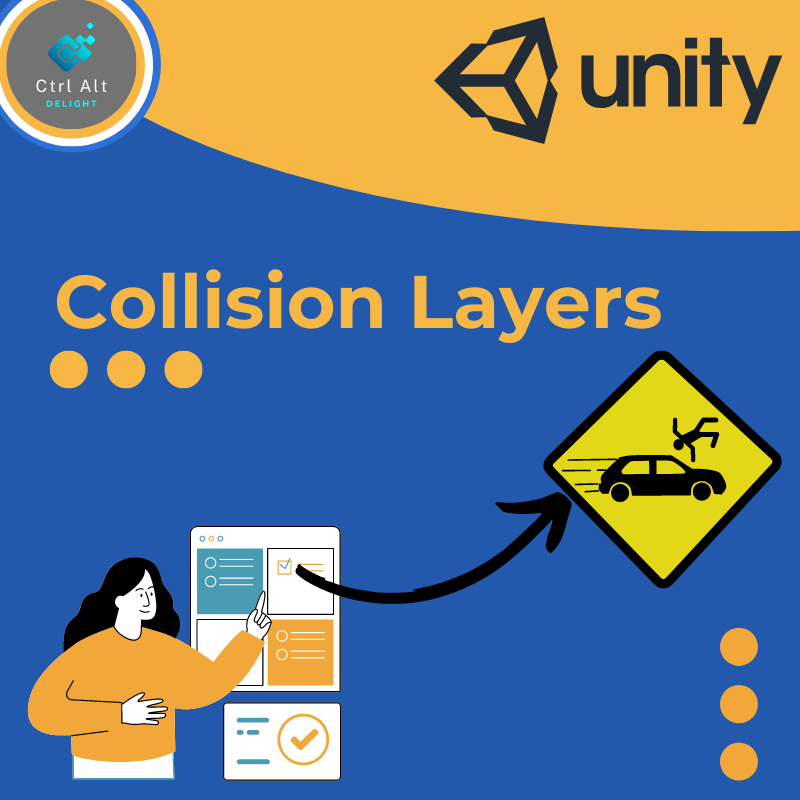
When handling collisions in a game, there comes a time when you don’t want everything to collide with everything else. To help you achieve this, you can use collision layers to define which layers collide with each other. In this post, I will explain the basics of collision layers.
Conclusion
In conclusion, collider events are an essential part of creating dynamic and interactive games in Unity. Understanding how to use them correctly can make a significant difference in the player’s experience. Whether it’s detecting collisions or triggering special effects, collider events can help bring your game to life.