Get ready to step up your game development skills with our crash course on the key functions in Unity! In this article, we’ll be breaking down the most important players in the game loop – Update, FixedUpdate, and LateUpdate. We’ll also give you the scoop on the crucial initialization and decommission functions, so you can get your objects up and running, and then shut ’em down with style. Whether you’re a seasoned pro or a newcomer to the game development scene, this guide is packed with all the info you need to take your skills to the next level. So buckle up, grab a coffee, and let’s dive into the Order of Update!
Unity Order of Execution Update life-cycle (short)
In the heart of the game loop, three functions take center stage. This quick guide will introduce you to these power players, show you how they work together, and explain what they’re used for. You’ll also get the inside scoop on how initialization and decommission functions fit into the mix.
- Update() is called once per frame. It is used for handling user inputs, performing animations and movements, and checking for collisions. It is called after the Start() function and before the LateUpdate() function.
- FixedUpdate() is used for physics calculations and is called at a fixed interval (i.e., it is not called every frame). This function is ideal for performing calculations related to physics, such as movement, rotation, and other physics-based interactions in your game.
- LateUpdate() is called after all other updates have been processed. It is used for tasks such as camera movements, ensuring objects are in their correct position, and making final adjustments to the game world. This function is ideal for performing calculations that depend on the updated state of the game world, as all other updates have already been processed.
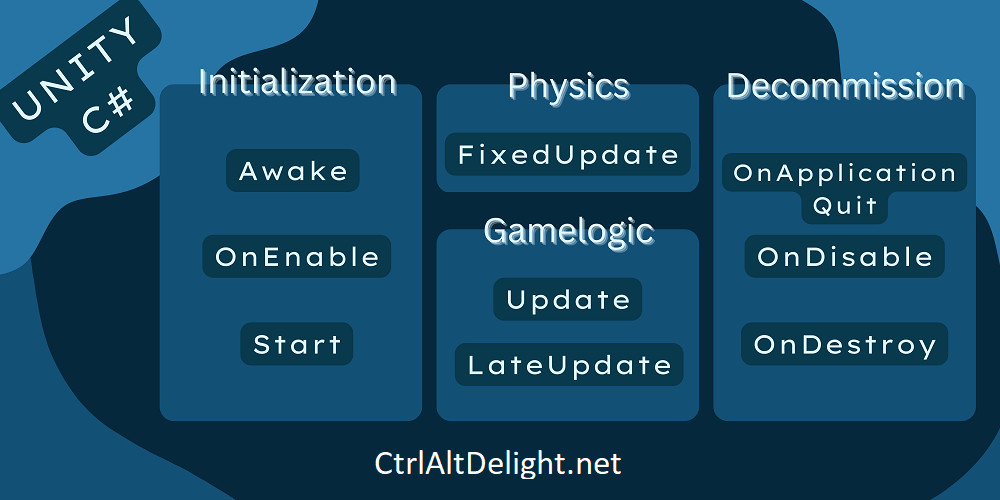
The FixedUpdate case
FixedUpdate’s got its own rhythm, it’s not following Update’s lead and keeps a steady beat with its fixed rate. Think of it as a metronome, while Update is a wild drummer who can’t stay on beat. In the chart below, you can see the typical scenario where FixedUpdate runs 50 times per second, that’s every 20 milliseconds, like a boss keeping time. Meanwhile, Update is running as fast as it can, limited by either the monitor’s refresh rate or the CPU’s power, like a drummer going crazy with 144 beats per second, that’s about every 7 milliseconds. As you can see, in the time FixedUpdate plays its one beat, Update jams out three beats!
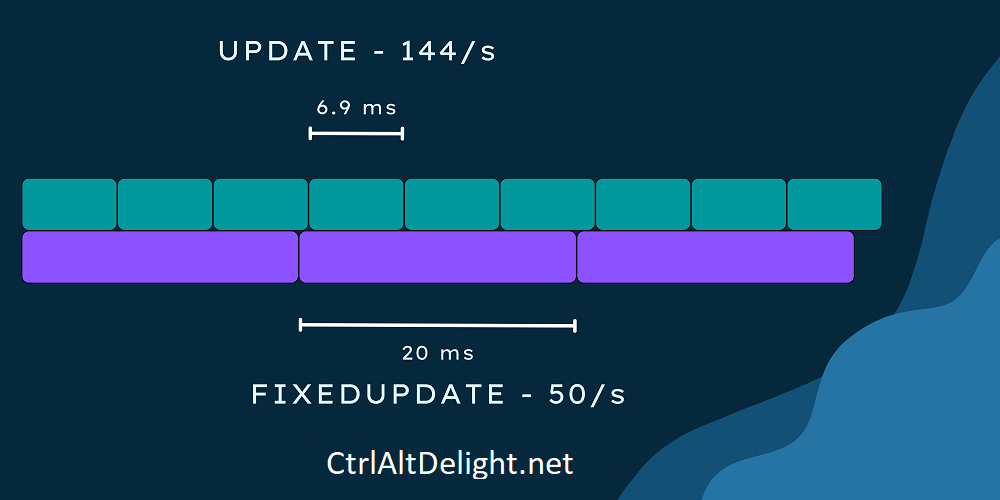
Initialization and Decommission
Initialization and decommission mark the beginning and end of an object’s lifespan in Unity. With a variety of events and functions at your disposal, you’re equipped to handle any situation that comes your way.
Initialization
During the initialization phase, you can equip your objects with the necessary information and connections. The three most commonly utilized initialization functions are:
- Awake() is called when an object is created, before any other initialization takes place. It is often used for initializing variables and setting up references between objects. The Awake() function is called before the Start() function.
- OnEnable() is called when an object is enabled, either when the object is created or after it has been disabled. This function is used for initializing an object’s state when it is enabled and can be used to set up any required dependencies.
- Start() is called after all objects have been initialized and is used for setting up an object’s initial state. This function is called after the Awake() function. It can be used to perform actions that depend on other objects having been fully initialized.
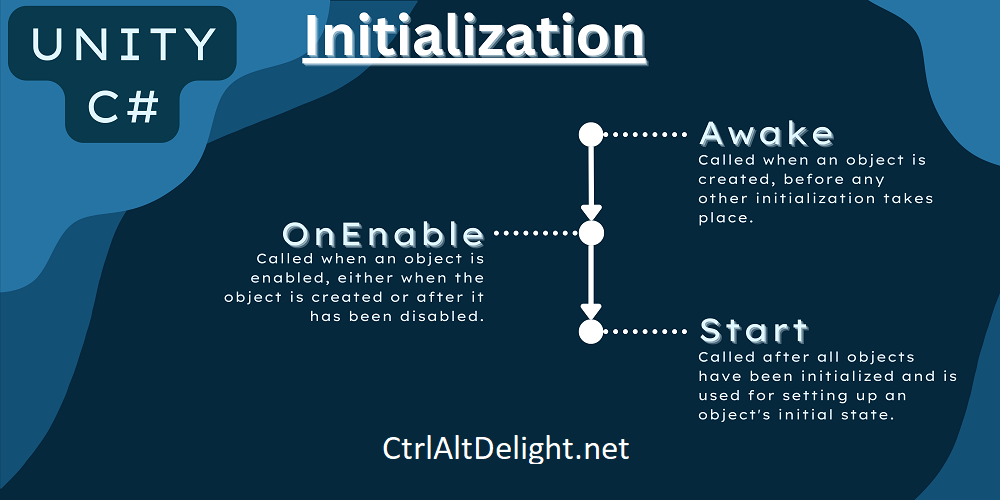
Decommission
The decommission phase lets you tidy up and maintain a pristine state for your game. The top three decommission functions in use are:
- OnApplicationQuit() is called when the application is quitting, either from the user or from an external system. It is used for cleaning up and saving data before the application exits.
- OnDisable() is called when an object is disabled. This happens either from a call to the gameObject.SetActive(false) method or from the object being destroyed. This function is used for cleaning up any resources associated with the object and for undoing any changes made by the OnEnable() function.
- OnDestroy() is called just before an object is destroyed and is used for freeing any resources associated with the object, such as releasing memory or breaking references to other objects.
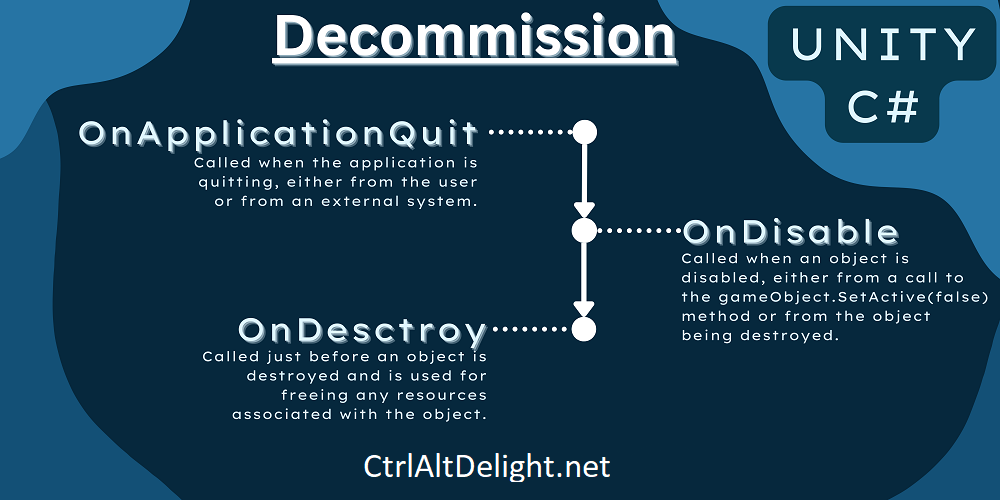
Further reading about Unity Order of Update
The Unity documentation is your best bud! For more in-depth info on this topic and all the extra functionalities in each phase, look no further than right here: ExecutionOrder (Unity doc)